Explicitly Access UxHw Functionality Using the UxHw API
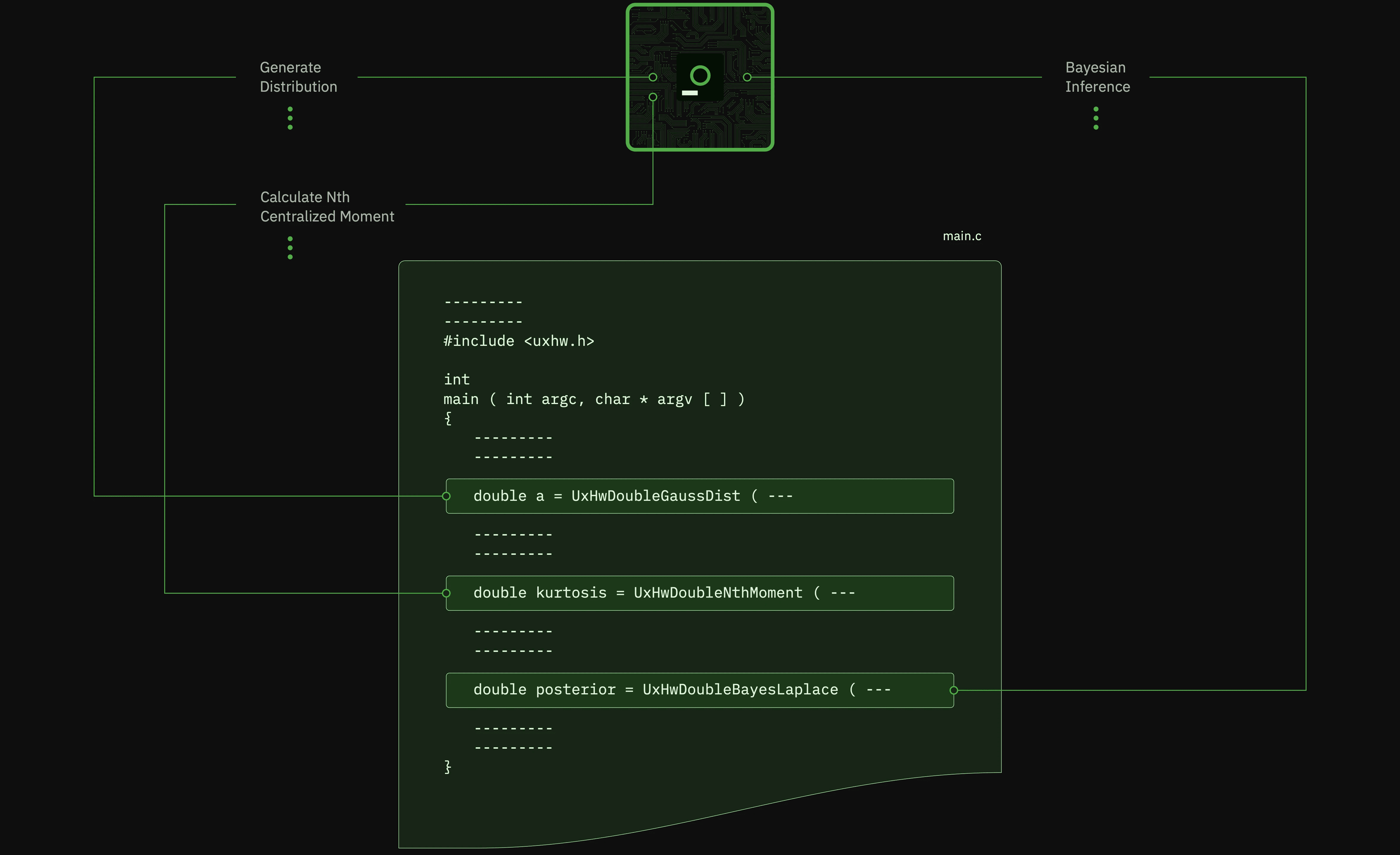
The Signaloid compute engine performs deterministic operations on representations of probability distributions (i.e., representations equivalent to a probability density function). In a program compiled for execution on either the cloud-based compute engine or the hardware modules, each value within a program has both its nominal value, e.g., 3.14, as well as a probability distribution around that number; informally, you can think of a probability distribution as being like a histogram with an arbitrary shape, potentially with multiple peaks. The plot below/right shows one example of a probability distribution around the number 3.14. The operations that the Signaloid compute engine performs on probability distributions are deterministic: Given two input values with associated distributions, an arithmetic operation running on the Signaloid compute engine will always lead to the same output distribution. This is in contrast to non-deterministic sampling-based methods such as Monte Carlo methods, where the calculated result distribution for some given number of Monte Carlo iterations (e.g., 1,000,000) will never lead to the exact same output distribution. We designed the programming model and architecture of the Signaloid compute engine to minimize the number of source code modifications (e.g., changes to variable types, linking against additional libraries, etc.) that an existing application would need to make to take advantage of the platform. The abstraction provided to software running over the Signaloid compute engine is that of a processor in which each individual single- or double-precision floating-point value also has an associated probability distribution within the microarchitecture below the exposed ISA abstraction. As a result, once values are fed into the processor with associated probability distribution information, applications do not need to explicitly call any functions in a library to take advantage of the Signaloid compute engine's updates of the distributions associated with values as program execution progresses. To allow the initialization of the distributions associated with inputs (and to query the distributions associated with outputs), the programming model provides the Uncertainty-Extended Hardware (UxHw) API, with functions for initializing, querying, and modifying distributional information of source code variables.
Figure 1: Example of a number with nominal value 3.14 as well as a probability distribution around that number.
Why It Matters
The UxHw API allows developers to programmatically initialize and query probability distributions that the underlying Signaloid compute engine associates, under the hood and not explicitly visible to programs, with every single value in a program. The functions of the UxHw API complement the ability of Signaloid's cloud-based and hardware compute engines to track data probability distributions through the operations of an application executable. Although the UxHw API functions are only necessary when programs need to either explicitly initialize probability distribution information in inputs or explicitly query the probability information associated with outputs, they form the foundation for creating new kinds of algorithms that introspect on the per-value probability distribution maintained by the Signaloid compute engine.
The Technical Details
Consider the program statement y = a * x + b;
. The UxHw API provides a way for a developer to explicitly initialize the distributions of a
, x
, and b
and to calculate statistics on the distribution y
. Applications can initialize the distribution information with parametric (i.e., analytic or "nameable") distributions such as Weibull, exponential, Bounded Pareto, Log-normal, Gaussian, Uniform, etc., or can initialize distributions using empirical data, correlated empirical joint distribution data or mixtures of distributions, to name but a few of the facilities. The code examples and plots at the end of this technology explainer give examples of these different APIs and their usage.
As one might expect, the UxHw API provides functions for computing summary statistics such as the moments of the distribution associated with a variable, modes, minimum support value and maximum support value values, tail probability, and quantiles as well as to obtain samples from a distribution. The carefully-thought-out structure of the UxHw programming model also allows applications to perform Bayesian inference: applications can use the Bayes-Laplace rule to calculate the probability of a hypothesis, given prior evidence, using the distributions tracked by the Signaloid compute engine. Again, the examples and plots at the end of this technology explainer give examples of these APIs and their usage.
Figure 2: An example of a distribution generated by calling the UxHw API function UxHwDoubleUniformDist to request a uniform distribution spanning -10 to 10.
The UxHw API also defines the uncertain extended/hexadecimal data format, a new, open, and well-documented format for representing probability distribution information. Applications can work with the Ux data format in two forms: Applications can process the Ux data format either as a string of characters which we refer to as a Ux Strings or as an array of unsigned bytes that we refer to as a Ux Byte Array. An example of a Ux String is:
0.785398Ux0400000000000000003FE921FB54442D18000000013FE921FB54442D188000000000000000
Ux Strings contain both traditional (point-valued or particle) numerical information (0.785398
in the example above) as well as uncertainty distribution information associated with that value (0400...0000
in the example above). The uncertainty information explicitly exploits knowledge of Signaloid's uncertainty tracking mathematics to represent both empirical as well as analytic/parametric distributions, in a discretized digital form. Applications can export the distributional information associated with one of their source code variables by simply using the printf()
family of functions of the C standard library, e.g., printf()
to print to the standard output stream of the application or fprintf()
to store the distributional information of a variable to a file. Applications can also read and ingest distributional information encoded as Ux strings using the scanf()
family of functions, e.g., fscanf()
to read a Ux string from a file in order to initialize the distributional information of a destination source code variable.
Relevant Code Example
Figure 3: An example of a distribution constructed from a small number of samples.
Figure 4: An example of a distribution created as a mixture of two Gaussians.
Figure 5: The variance of the mixture distribution of Figure 4 is a degenerate distribution (a particle), i.e., a value with no uncertainty.
The Takeaway
The UxHw API provides an explicit way to access the Signaloid compute engine's probability distribution arithmetic and probability distribution data representations. Applications can use the UxHw API to initialize the probability distributions of variables in the source code, query their statistical properties, and calculate the probability of them taking a range of values. The UxHw API also defines the Ux Data format, a new, open, and well-documented interchange format for representing both analytic and empirical probability distributions.